Ma az Android Fundamentals 02.2: Activity lifecycle and state fejezetet vettem át, az alkalmazások életciklusát vettük át a naplózás segítségével. Bár erről már volt szó Tim Bulchalka Android Java Masterclass kurzusán, leírva jobban megértettem. Tim jó sokat beszélt az egészről és a videón elsikkadt a lényeg.
Az alábbi kép az Android Fundamentals-ból származik, és ezt próbálgattam a TwoActivities alkalmazás bővítésével. Az alapból felülírt onCreate() metódus mellett felülírtam a MainActivity-ben és a SecondActivity-ben az onStart(), onPause(), onRestart(), onResume(), onStop() és onDestroy() metódusokat, és az alkalmazást futtatva a Logcat naplópanelen néztem a történteket. Érdekes volt látni, hogyan működnek ezek a folyamatok az activity-k közötti váltáskor.

Ebben a fejezetben volt egy programozási feladat, az elmúlt néhány órában ezen dolgoztam:
Challenge: Create a simple shopping-list app with a main activity for the list the user is building, and a second activity for a list of common shopping items.
- The main activity should contain the list to build, which should be made up of ten empty
TextView
elements. - An Add Item button on the main activity launches a second activity that contains a list of common shopping items (Cheese, Rice, Apples, and so on). Use
Button
elements to display the items. - Choosing an item returns the user to the main activity, and updates an empty
TextView
to include the chosen item.
Use an Intent
to pass information from one Activity
to another. Make sure that the current state of the shopping list is saved when the user rotates the device.
Azaz, csináltam egy bevásárlólista-alkalmazást, ahol a kezdő képernyőn 10 hely volt a termékeknek. Egy Add Item gomb megnyomásával kerültem a második képernyőre, itt pedig előre felvitt termékek voltak gombként. Én 21 gombot tettem fel. A gombot megnyomva visszakerültünk a főképernyőre, és sorban elkezdtem feltölteni a 10 helyet a kiválasztott termékekkel. Ha már tele volt, akkor én úgy döntöttem, hogy a 10. helyen lévő terméket cserélem le újra és újra.
A layoutok elkészítése
LinearLayout-ot használtam az elemek elrendezésére. Az activity_second.xml fájlban mindegyik sor egy-egy LinearLayout volt, amely vízszintesen rendezte el az elemeket. 3 gombot raktam egy sorba, így lett 7 sorom. Ezt a 7 darab LinerLayout-ot közre fogtam egy szülő LinearLayout-tal, mely függőlegesen elrendezte a sorokat.
Az activity_main.xml fájlban ConstraintLayout-ot használtam, a 10 sort a képernyő tetejéhez igazítottam, a gombot pedig az aljához.
Elkészítettem a fektetett, landscape verziókat is, itt viszont már a főképernyőn sem fért ki egymás alá a 10 termék helye, ezért kettesével sorokba rendeztem őket, alulra a gombbal. A második képernyő landscape verziójánál pedig több gombot tettem egy sorba. Ez a layout-buherálás jó dolog volt, mert kezdem megszokni a különböző layoutok használatát.
A két Java fájl
Az Add Item gombra felfűztem egy launchSecondActivity metódust, amelynek semmilyen más feladata nem volt, mint egy eredményt kérő Intentet küldeni a SecondActivity.java-nak.
public void launchSecondActivity(View view) { Intent intent = new Intent(this, SecondActivity.class); startActivityForResult(intent, PRODUCT_REQUEST); }
A SecondActivity élőre tette a activity_second.xml második képernyőt, ahol a gombok mindegyikére felfűztem a getProduct metódust. Nagyon jó dolog, hogy az onClick-kel át tudja adni a Java az őt küldő View-t, így nem kellett a 21 gombnak 21 metódust írnom. Egyszerűen Button típusra kasztoltam a View-t, majd az Intenttel visszaküldtem a Main Activity-nak a gomb szövegét.
public void getProduct(View view) { Button button = (Button) view; Intent replyIntent = new Intent(); replyIntent.putExtra(PRODUCT, button.getText().toString()); setResult(RESULT_OK, replyIntent); finish(); }
A visszatérő eredmény aktiválta a MainActivity.java-ban az onActivityResult() metódust, ahol kinyertem a küldő gomb szövegét (ami ugye a termék neve), majd ezt átadtam egy belső fillList() metódusnak. Ebben vizsgáltam egy belső változó értékét, melyet 1 kezdőértékkel határoztam meg. Ez mindig azt mutatta, hányadik helyre kell betenni a megkapott terméknevet a bevásárlólistán. Ha betelt, akkor már csak a 10. helyen cserélgettem a termékneveket.
Ez volt kb. a feladat, emellett már csak azt kellett megoldanom, hogy a forgatáskor meglévő adatokat vissza tudjam állítani a Bundle-ből. Az XML fájlokból pedig az értékeket és a neveket szépen kiexportáltam külön XML-ekbe, ami jól is jött, amikor a landscape verziónál változtatnom kellett a betűméreteket. Így egy helyen kellett átírni, és nem mind a 21 gombnál külön-külön.
A termékneveket is exportáltam a strings.xmnl fájlba, így akár le is lehet fordítani az alkalmazást 2 perc alatt.
Képernyőképek
Az alkalmazás indulás után portrait és landscape módban
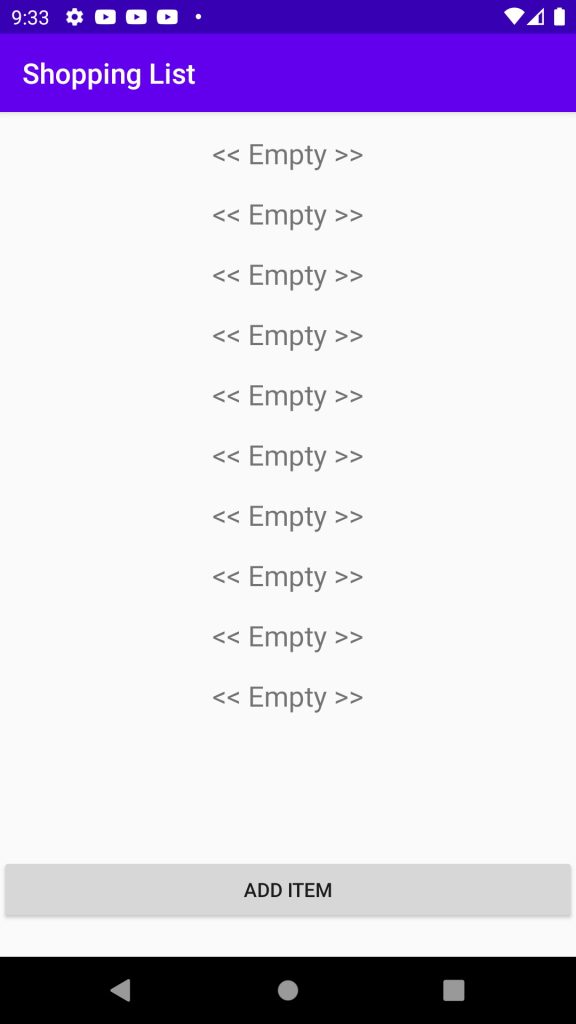
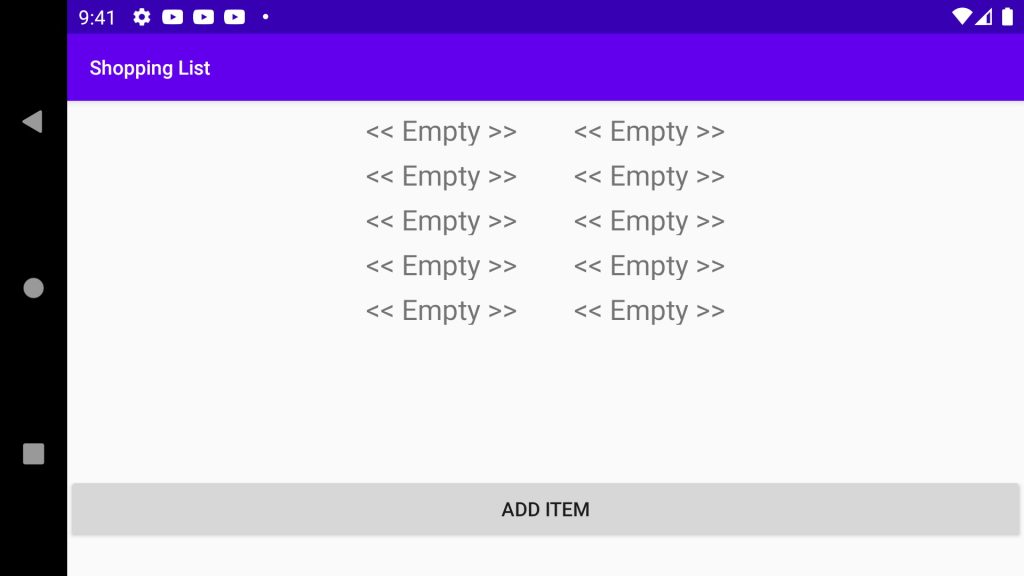
A terméknevek portrait és landscape módban
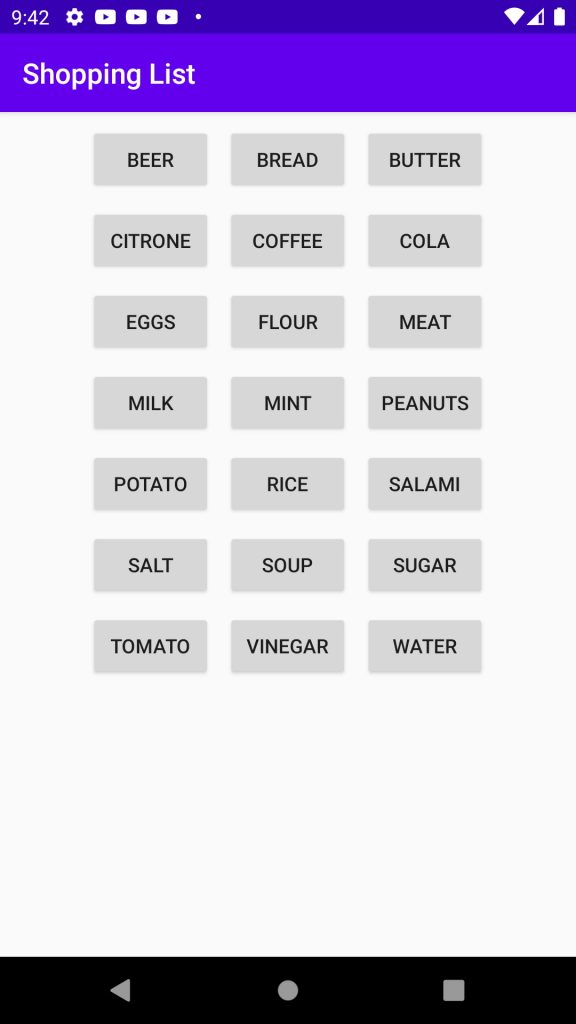
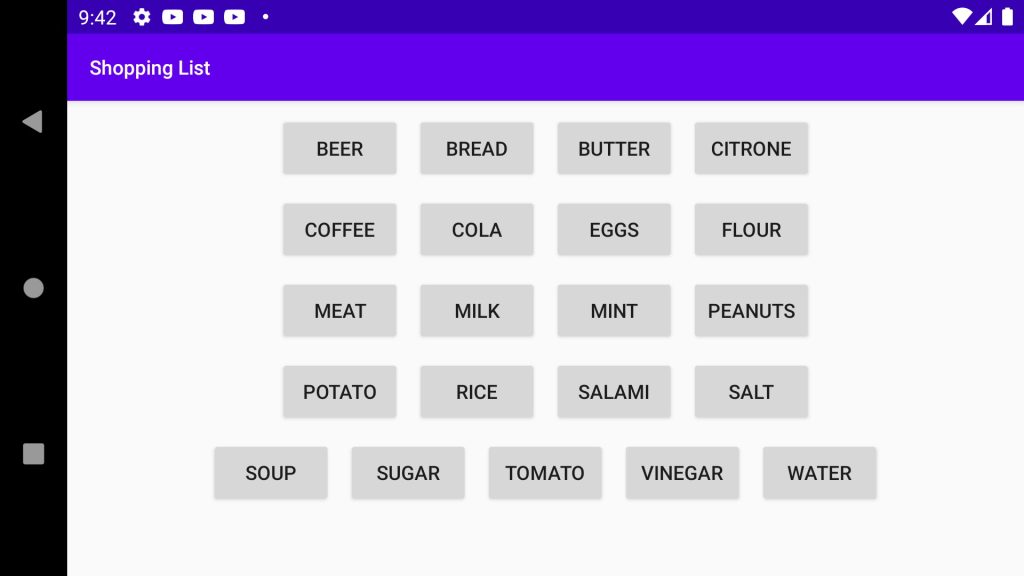
A feltöltött lista portrait és landscape módban
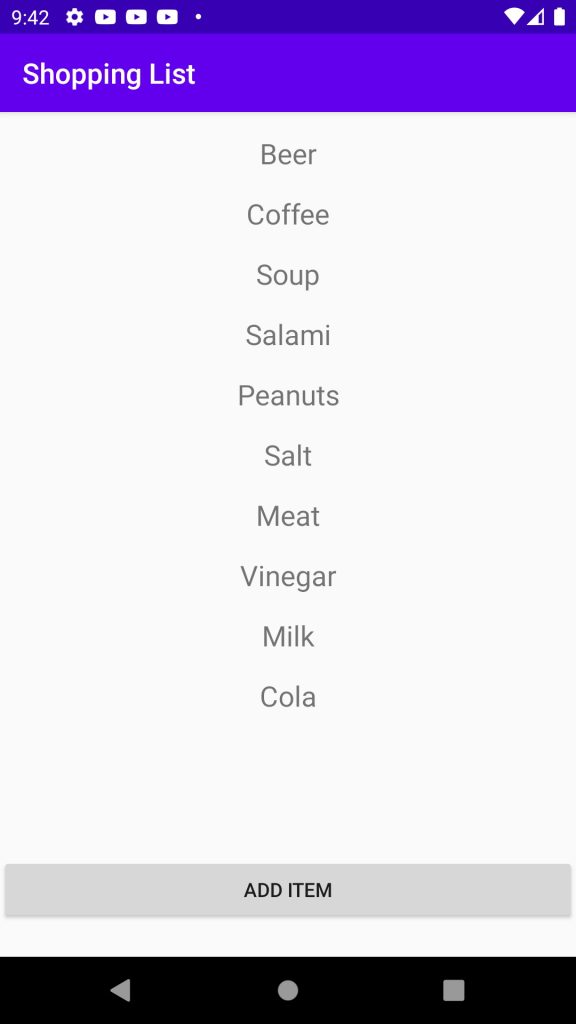
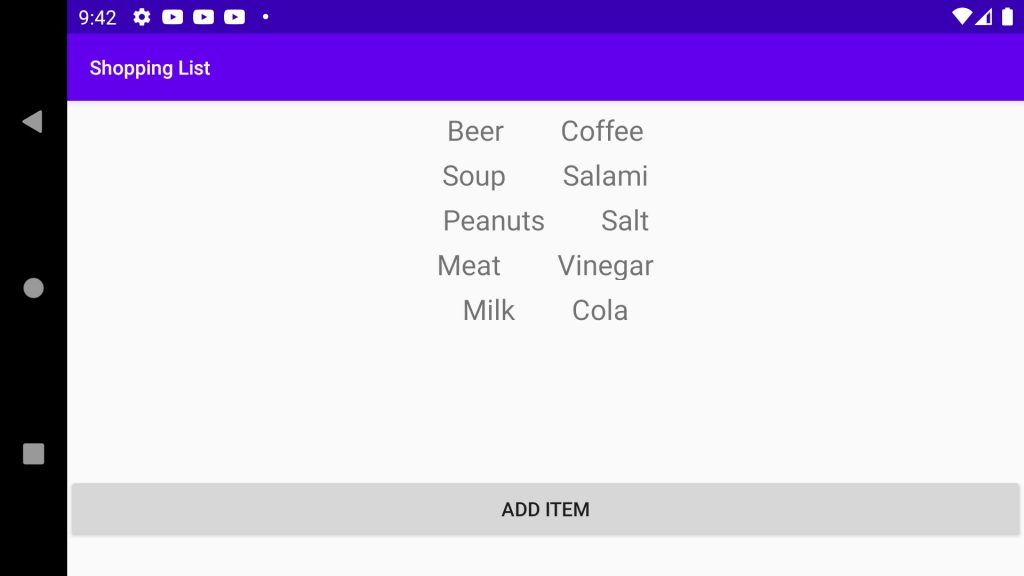
Forráskód
layout/activity_main.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <TextView android:id="@+id/textView1" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent" /> <TextView android:id="@+id/textView2" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/textView1" /> <TextView android:id="@+id/textView3" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/textView2" /> <TextView android:id="@+id/textView4" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/textView3" /> <TextView android:id="@+id/textView5" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/textView4" /> <TextView android:id="@+id/textView6" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/textView5" /> <TextView android:id="@+id/textView7" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/textView6" /> <TextView android:id="@+id/textView8" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/textView7" /> <TextView android:id="@+id/textView9" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/textView8" /> <TextView android:id="@+id/textView10" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toBottomOf="@+id/textView9" /> <Button android:id="@+id/button" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginBottom="@dimen/layout_margin_bottom" android:onClick="launchSecondActivity" android:text="@string/add_item" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" /> </androidx.constraintlayout.widget.ConstraintLayout>
layout/activity_second.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:orientation="vertical" tools:context=".SecondActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_second" android:gravity="center" android:orientation="horizontal"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product1" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product2" /> <Button android:id="@+id/button3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product3" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_second" android:gravity="center" android:orientation="horizontal"> <Button android:id="@+id/button4" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product4" /> <Button android:id="@+id/button5" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product5" /> <Button android:id="@+id/button6" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product6" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_second" android:gravity="center" android:orientation="horizontal"> <Button android:id="@+id/button7" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product7" /> <Button android:id="@+id/button8" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product8" /> <Button android:id="@+id/button9" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product9" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_second" android:gravity="center" android:orientation="horizontal"> <Button android:id="@+id/button10" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product10" /> <Button android:id="@+id/button11" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product11" /> <Button android:id="@+id/button12" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product12" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_second" android:gravity="center" android:orientation="horizontal"> <Button android:id="@+id/button13" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product13" /> <Button android:id="@+id/button14" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product14" /> <Button android:id="@+id/button15" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product15" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_second" android:gravity="center" android:orientation="horizontal"> <Button android:id="@+id/button16" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product16" /> <Button android:id="@+id/button17" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product17" /> <Button android:id="@+id/button18" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product18" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_second" android:gravity="center" android:orientation="horizontal"> <Button android:id="@+id/button19" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product19" /> <Button android:id="@+id/button20" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product20" /> <Button android:id="@+id/button21" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_second" android:layout_marginRight="@dimen/layout_margin_right_second" android:onClick="getProduct" android:text="@string/product21" /> </LinearLayout> </LinearLayout>
land/acitivy_main.xml
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".MainActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:orientation="horizontal"> <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_land" android:layout_marginRight="@dimen/layout_margin_right_land" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size_land" /> <TextView android:id="@+id/textView2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land" android:layout_marginTop="@dimen/layout_margin_top_land" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size_land" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:orientation="horizontal"> <TextView android:id="@+id/textView3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_land" android:layout_marginRight="@dimen/layout_margin_right_land" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size_land" /> <TextView android:id="@+id/textView4" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land" android:layout_marginTop="@dimen/layout_margin_top_land" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size_land" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:orientation="horizontal"> <TextView android:id="@+id/textView5" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_land" android:layout_marginRight="@dimen/layout_margin_right_land" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size_land" /> <TextView android:id="@+id/textView6" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land" android:layout_marginTop="@dimen/layout_margin_top_land" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size_land" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:orientation="horizontal"> <TextView android:id="@+id/textView7" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_land" android:layout_marginRight="@dimen/layout_margin_right_land" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size_land" /> <TextView android:id="@+id/textView8" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land" android:layout_marginTop="@dimen/layout_margin_top_land" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size_land" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:orientation="horizontal"> <TextView android:id="@+id/textView9" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_land" android:layout_marginRight="@dimen/layout_margin_right_land" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size_land" /> <TextView android:id="@+id/textView10" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land" android:layout_marginTop="@dimen/layout_margin_top_land" android:gravity="center" android:text="@string/empty_label" android:textSize="@dimen/text_size_land" /> </LinearLayout> </LinearLayout> <Button android:id="@+id/button" android:layout_width="0dp" android:layout_height="wrap_content" android:layout_marginBottom="@dimen/layout_margin_bottom_land" android:onClick="launchSecondActivity" android:text="Add Item" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" /> </androidx.constraintlayout.widget.ConstraintLayout>
land/activity_second.xml
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="wrap_content" android:gravity="center" android:orientation="vertical" tools:context=".SecondActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_land_second" android:gravity="center" android:orientation="horizontal"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product1" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product2" /> <Button android:id="@+id/button3" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product3" /> <Button android:id="@+id/button4" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product4" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_land_second" android:gravity="center" android:orientation="horizontal"> <Button android:id="@+id/button5" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product5" /> <Button android:id="@+id/button6" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product6" /> <Button android:id="@+id/button7" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product7" /> <Button android:id="@+id/button8" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product8" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_land_second" android:gravity="center" android:orientation="horizontal"> <Button android:id="@+id/button9" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product9" /> <Button android:id="@+id/button10" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product10" /> <Button android:id="@+id/button11" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product11" /> <Button android:id="@+id/button12" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product12" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_land_second" android:gravity="center" android:orientation="horizontal"> <Button android:id="@+id/button13" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product13" /> <Button android:id="@+id/button14" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product14" /> <Button android:id="@+id/button15" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product15" /> <Button android:id="@+id/button16" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product16" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginTop="@dimen/layout_margin_top_land_second" android:gravity="center" android:orientation="horizontal"> <Button android:id="@+id/button17" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product17" /> <Button android:id="@+id/button18" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product18" /> <Button android:id="@+id/button19" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product19" /> <Button android:id="@+id/button20" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product20" /> <Button android:id="@+id/button21" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_marginLeft="@dimen/layout_margin_left_land_second" android:layout_marginRight="@dimen/layout_margin_right_land_second" android:onClick="getProduct" android:text="@string/product21" /> </LinearLayout> </LinearLayout>
dimens.xml
<?xml version="1.0" encoding="utf-8"?> <resources> <dimen name="layout_margin_top">16dp</dimen> <dimen name="text_size">20sp</dimen> <dimen name="layout_margin_bottom">24dp</dimen> <dimen name="layout_margin_top_land">5dp</dimen> <dimen name="layout_margin_right_land">20dp</dimen> <dimen name="layout_margin_left_land">20dp</dimen> <dimen name="text_size_land">20dp</dimen> <dimen name="layout_margin_bottom_land">24dp</dimen> <dimen name="layout_margin_top_second">10dp</dimen> <dimen name="layout_margin_left_second">5dp</dimen> <dimen name="layout_margin_right_second">5dp</dimen> <dimen name="layout_margin_top_land_second">10dp</dimen> <dimen name="layout_margin_left_land_second">5dp</dimen> <dimen name="layout_margin_right_land_second">5dp</dimen> </resources>
strings.xml
<resources> <string name="app_name">Shopping List</string> <string name="empty_label"><![CDATA[<< Empty >>]]></string> <string name="add_item">Add Item</string> <string name="product1">Beer</string> <string name="product2">Bread</string> <string name="product3">Butter</string> <string name="product4">Citrone</string> <string name="product5">Coffee</string> <string name="product6">Cola</string> <string name="product7">Eggs</string> <string name="product8">Flour</string> <string name="product9">Meat</string> <string name="product10">Milk</string> <string name="product11">Mint</string> <string name="product12">Peanuts</string> <string name="product13">Potato</string> <string name="product14">Rice</string> <string name="product15">Salami</string> <string name="product16">Salt</string> <string name="product17">Soup</string> <string name="product18">Sugar</string> <string name="product19">Tomato</string> <string name="product20">Vinegar</string> <string name="product21">Water</string> </resources>
MainActivity.java
package com.viktorjava.shoppinglist; import androidx.annotation.NonNull; import androidx.annotation.Nullable; import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.TextView; public class MainActivity extends AppCompatActivity { private static final String TAG = "TAG"; private TextView tw1; private TextView tw2; private TextView tw3; private TextView tw4; private TextView tw5; private TextView tw6; private TextView tw7; private TextView tw8; private TextView tw9; private TextView tw10; public static final int PRODUCT_REQUEST = 1; private int nr = 1; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); tw1 = (TextView) findViewById(R.id.textView1); tw2 = (TextView) findViewById(R.id.textView2); tw3 = (TextView) findViewById(R.id.textView3); tw4 = (TextView) findViewById(R.id.textView4); tw5 = (TextView) findViewById(R.id.textView5); tw6 = (TextView) findViewById(R.id.textView6); tw7 = (TextView) findViewById(R.id.textView7); tw8 = (TextView) findViewById(R.id.textView8); tw9 = (TextView) findViewById(R.id.textView9); tw10 = (TextView) findViewById(R.id.textView10); if (savedInstanceState != null) { tw1.setText(savedInstanceState.getString("product1")); tw2.setText(savedInstanceState.getString("product2")); tw3.setText(savedInstanceState.getString("product3")); tw4.setText(savedInstanceState.getString("product4")); tw5.setText(savedInstanceState.getString("product5")); tw6.setText(savedInstanceState.getString("product6")); tw7.setText(savedInstanceState.getString("product7")); tw8.setText(savedInstanceState.getString("product8")); tw9.setText(savedInstanceState.getString("product9")); tw10.setText(savedInstanceState.getString("product10")); nr = savedInstanceState.getInt("nr"); } } public void launchSecondActivity(View view) { Intent intent = new Intent(this, SecondActivity.class); startActivityForResult(intent, PRODUCT_REQUEST); } @Override protected void onActivityResult(int requestCode, int resultCode, @Nullable Intent data) { super.onActivityResult(requestCode, resultCode, data); if (requestCode == PRODUCT_REQUEST) { if (resultCode == RESULT_OK) { fillList(data.getStringExtra(SecondActivity.PRODUCT)); } } } @Override protected void onSaveInstanceState(@NonNull Bundle outState) { outState.putString("product1", tw1.getText().toString()); outState.putString("product2", tw2.getText().toString()); outState.putString("product3", tw3.getText().toString()); outState.putString("product4", tw4.getText().toString()); outState.putString("product5", tw5.getText().toString()); outState.putString("product6", tw6.getText().toString()); outState.putString("product7", tw7.getText().toString()); outState.putString("product8", tw8.getText().toString()); outState.putString("product9", tw9.getText().toString()); outState.putString("product10", tw10.getText().toString()); outState.putInt("nr", nr); super.onSaveInstanceState(outState); } private void fillList(String product) { switch (nr) { case 1: tw1.setText(product); nr++; break; case 2: tw2.setText(product); nr++; break; case 3: tw3.setText(product); nr++; break; case 4: tw4.setText(product); nr++; break; case 5: tw5.setText(product); nr++; break; case 6: tw6.setText(product); nr++; break; case 7: tw7.setText(product); nr++; break; case 8: tw8.setText(product); nr++; break; case 9: tw9.setText(product); nr++; break; default: tw10.setText(product); break; } } }
SecondActivity.java
package com.viktorjava.shoppinglist; import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; import android.view.View; import android.widget.Button; public class SecondActivity extends AppCompatActivity { public static final String PRODUCT = ".SecondActivity.PRODUCT"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_second); } public void getProduct(View view) { Button button = (Button) view; Intent replyIntent = new Intent(); replyIntent.putExtra(PRODUCT, button.getText().toString()); setResult(RESULT_OK, replyIntent); finish(); } }
Megoldandó feladatok
Nem tetszik az igazítás a landscape verzió bevásárlólistájában, az sem nem középre igazított, sem nem valamelyik széléhez. Az álló verzióban érdekes, nincs hiba. Nem túl nagy probléma, de érdekel, ezért igyekszem kinyomozni.
Továbbá az applikáció most nem figyeli, hozzá van-e adva egy elem már a bevásálólistához. Figyelhetné, és egy Toast üzenetben dobhatna hibát újbóli hozzáadáskor.
Felhasznált segédletek
- Android fundamentals 02.2: Activity lifecycle and state
- How do I center text horizontally and vertically in a TextView?
- Android – How to set padding for TextView in ListView or ExpandableListView
- Android Developers Guides – Relative Layout
- Android Developers Reference – LinearLayout
- How to make space between LinearLayout children?
Vélemény, hozzászólás?