Az előző bejegyzésben bemutatott feladat szorgalmi volt, az Android fundamentals 02.1: Activities and intents fejezet igazi házi feladata ez volt:
Open the HelloToast app that you created in a previous practical codelab.
- Modify the Toast button so that it launches a new
Activity
to display the word „Hello!” and the current count, as shown below. - Change the text on the
Toast
button to Say Hello.
A HelloToast alkalmazás arról szól, hogy volt egy gomb az alkalmazás tetején, ha ezt megnyomtuk, akkor a képernyő aljára egy Toast üzenetet írt ki, hogy „Hello Toast!”. Alatta volt egy számláló, aminek az értékét a képernyő alján helyet kapó másik gombbal lehetett növelni. Így nézett ki:

Na a házi feladatban ezt az alkalmazást kellett módosítani, hogy a Toast gomb helyett egy Say Hello gomb legyen, amit megnyomva az új Activity-nek küldje át a számláló aktuális állását. A SecondActivity-ben ki kellett írni, hogy Say Hello! és alája az értéket.
A második Activity kinézetét egy LinearLayout beszúrásával oldottam meg, ezzel hoztam középre a két TextView-t. Ezeket a Layoutokat még gyakorolni kell, mert bár működik az app, nem vagyok benne biztos, hogy minden tökéletes az XML fájlban.
Az Intent átadásával is megszenvedtem, mert most nem Stringet küldtem át a SecondActivity-nek, hanem int értéket, és szépen át is ment, de a fogadó oldalon nem tudtam kinyerni. Folyamatosan null értéket kaptam. A hiba itt volt:
counterViewView.setText(Integer.toString(intent.getIntExtra("COUNT", 0)));
Nem adtam meg a kontextust, erre egy óra után rá is jöttem 🙂 A helyes megoldás:
counterViewView.setText(Integer.toString(intent.getIntExtra(MainActivity.COUNT, 0)));
Innentől fogva már csak egy kis formázás kellett a második Activity két TextView-jára, és már ment is minden, mint a karikacsapás.
Képernyőképek
Így indult az alkalmazás:
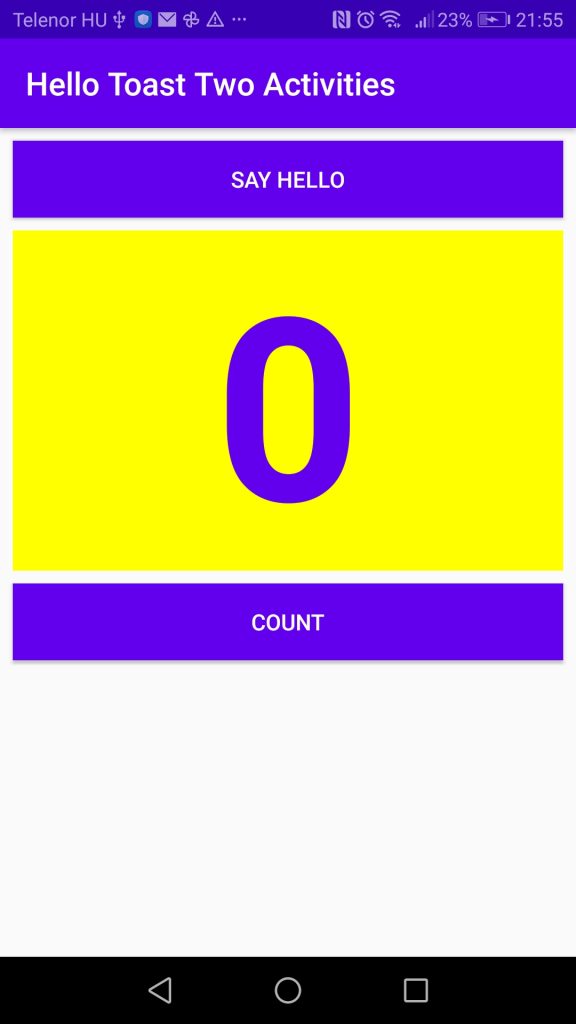
Ezt követően párszor megnyomtam a Count gombot, hogy növeljem a számláló értékét:
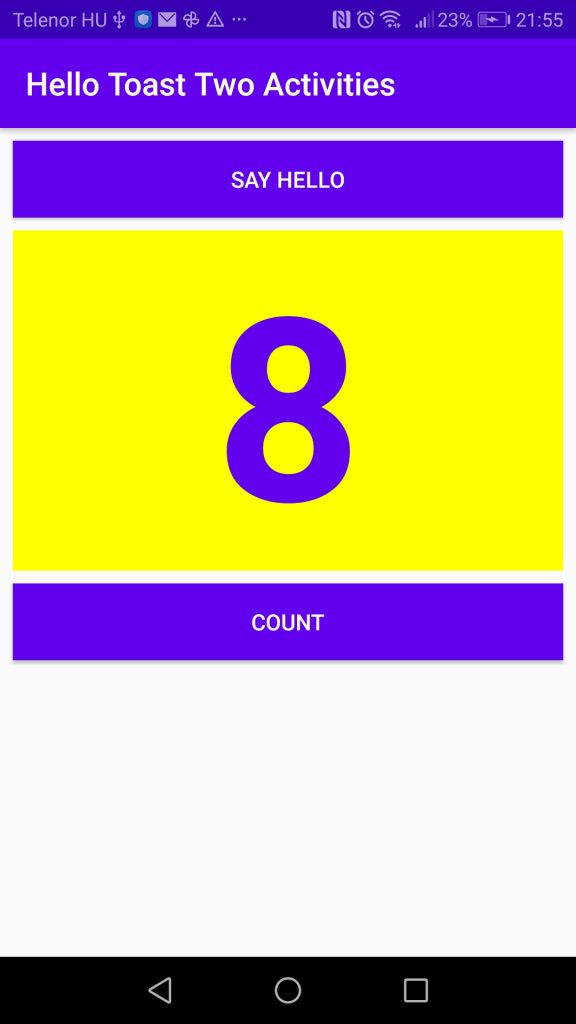
Végül pedig a Say Hello gomb megnyomásával átküldtem a Second Acticity-nek a számláló értékét, és kiírtam a köszöntést:
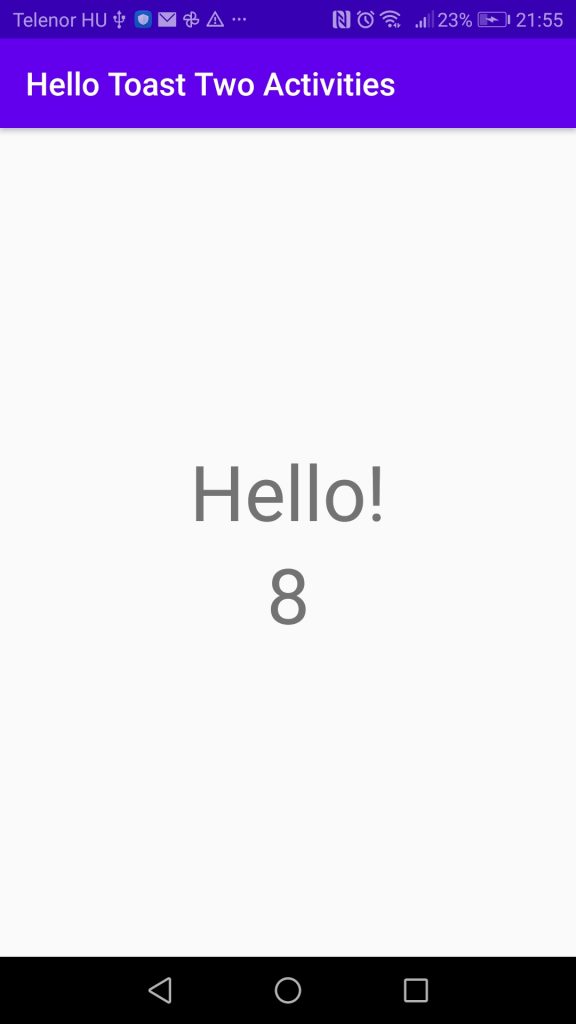
Forráskód
activity_main.xml:
<?xml version="1.0" encoding="utf-8"?> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:orientation="vertical" tools:context=".MainActivity"> <Button android:id="@+id/button_toast" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:layout_marginEnd="8dp" android:background="@color/colorPrimary" android:onClick="openToast" android:text="@string/button_label_toast" android:textColor="@android:color/white" /> <TextView android:id="@+id/show_count" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@+id/button_toast" android:layout_alignParentStart="true" android:layout_alignParentLeft="true" android:layout_marginStart="8dp" android:layout_marginTop="8dp" android:layout_marginEnd="8dp" android:layout_marginBottom="8dp" android:background="#FFFF00" android:gravity="center_vertical" android:text="@string/count_initial_value" android:textAlignment="center" android:textColor="@color/colorPrimary" android:textSize="160sp" android:textStyle="bold" /> <Button android:id="@+id/button_count" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_below="@+id/show_count" android:layout_centerHorizontal="true" android:layout_marginStart="8dp" android:layout_marginEnd="8dp" android:layout_marginBottom="8dp" android:background="@color/colorPrimary" android:onClick="countUp" android:text="@string/button_label_count" android:textColor="@android:color/white" /> </RelativeLayout>
strings.xml:
<resources> <string name="app_name">Hello Toast Two Activities</string> <string name="button_label_toast">Say Hello</string> <string name="button_label_count">Count</string> <string name="count_initial_value">0</string> <string name="toast_message">Hello Toast!</string> </resources>
MainActivity.java:
package com.viktorjava.hellotoast; import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.TextView; import android.widget.Toast; public class MainActivity extends AppCompatActivity { private static final String TAG = "TAG"; private int mCount = 0; private TextView mShowCount; public static final String COUNT = "com.viktorjava.android.hellotoastwoactivities.COUNT"; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); mShowCount = (TextView) findViewById(R.id.show_count); } public void showToast(View view) { Toast toast = Toast.makeText(this, R.string.toast_message, Toast.LENGTH_SHORT); toast.show(); } public void countUp(View view) { mCount++; if (mShowCount != null) mShowCount.setText(Integer.toString(mCount)); } public void openToast(View view) { Intent intent = new Intent(this, SecondActivity.class); intent.putExtra(COUNT, Integer.parseInt(mShowCount.getText().toString())); startActivity(intent); } }
activity_second.xml:
<?xml version="1.0" encoding="utf-8"?> <androidx.constraintlayout.widget.ConstraintLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" tools:context=".SecondActivity"> <LinearLayout android:layout_width="wrap_content" android:layout_height="wrap_content" android:orientation="vertical" app:layout_constraintBottom_toBottomOf="parent" app:layout_constraintEnd_toEndOf="parent" app:layout_constraintStart_toStartOf="parent" app:layout_constraintTop_toTopOf="parent"> <TextView android:id="@+id/helloView" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="1" android:gravity="center" android:textAlignment="center" android:textSize="48dp" /> <TextView android:id="@+id/counterView" android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_gravity="center_vertical" android:layout_weight="1" android:gravity="center" android:textAlignment="center" android:textSize="48dp" /> </LinearLayout> </androidx.constraintlayout.widget.ConstraintLayout>
SecondActivity.java:
package com.viktorjava.hellotoast; import androidx.appcompat.app.AppCompatActivity; import android.content.Intent; import android.os.Bundle; import android.util.Log; import android.widget.TextView; public class SecondActivity extends AppCompatActivity { private static final String TAG = "TAG"; TextView helloViewView; TextView counterViewView; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_second); helloViewView = (TextView) findViewById(R.id.helloView); counterViewView = (TextView) findViewById(R.id.counterView); Intent intent = getIntent(); helloViewView.setText("Hello!"); counterViewView.setText(Integer.toString(intent.getIntExtra(MainActivity.COUNT, 0))); } }
Vélemény, hozzászólás?